Yuha on Aug 17, 20222022-08-17T15:00:00+09:00
Updated Aug 17, 20222022-08-17T18:15:00+09:00 4 min read
What is Swagger?
1
| one of tools for describing RESTful APIs by OpenAPI specification
|
- OAS (OpenAPI Specification)
- the way to express RESTful API in json or yaml according to predefined rules
- the ways to visualize Swagger
- Swagger UI
- lets you edit OpenAPI specifications in YAML inside your browser and to preview documentations in real time.
- Swagger Editor
- a collection of HTML, Javascript, and CSS assets that dynamically generate beautiful documentation from an OAS-compliant API.
- Swagger Codegen
- code generator. You can use it to generate server stubs and client SDKs from an OpenAPI definition.
- reference :
https://gruuuuu.github.io/programming/openapi/
https://swagger.io/specification/
Benefits
- human readable and machine readable API documentation
- Swagger allows you to describe the structure of your APIs, so that machines can read them.
- easily adjustable
- interactive
How to use Swagger
springdoc-openapi vs springfox-swagger
Springdoc
- recently, commonly use
Swagger3
- a much more recent library that does not have so much legacy code as Springfox
Springfox
- deprecated
- The project seems to be no longer maintained
- last commit is of Oct 14, 2020
Springdoc
build.gradle
1
| implementation 'org.springdoc:springdoc-openapi-ui:1.5.10'
|
application.yml
1
2
3
| springdoc:
swagger-ui:
path: /swagger-ui.html
|
Annotations
https://jeonyoungho.github.io/posts/Open-API-3.0-Swagger-v3-%EC%83%81%EC%84%B8%EC%84%A4%EC%A0%95/
1
| import io.swagger.v3.oas.annotations.*;
|
@Tag
Example
1
| @Tag(name = "Item", description = "ํ๋ชฉ")
|
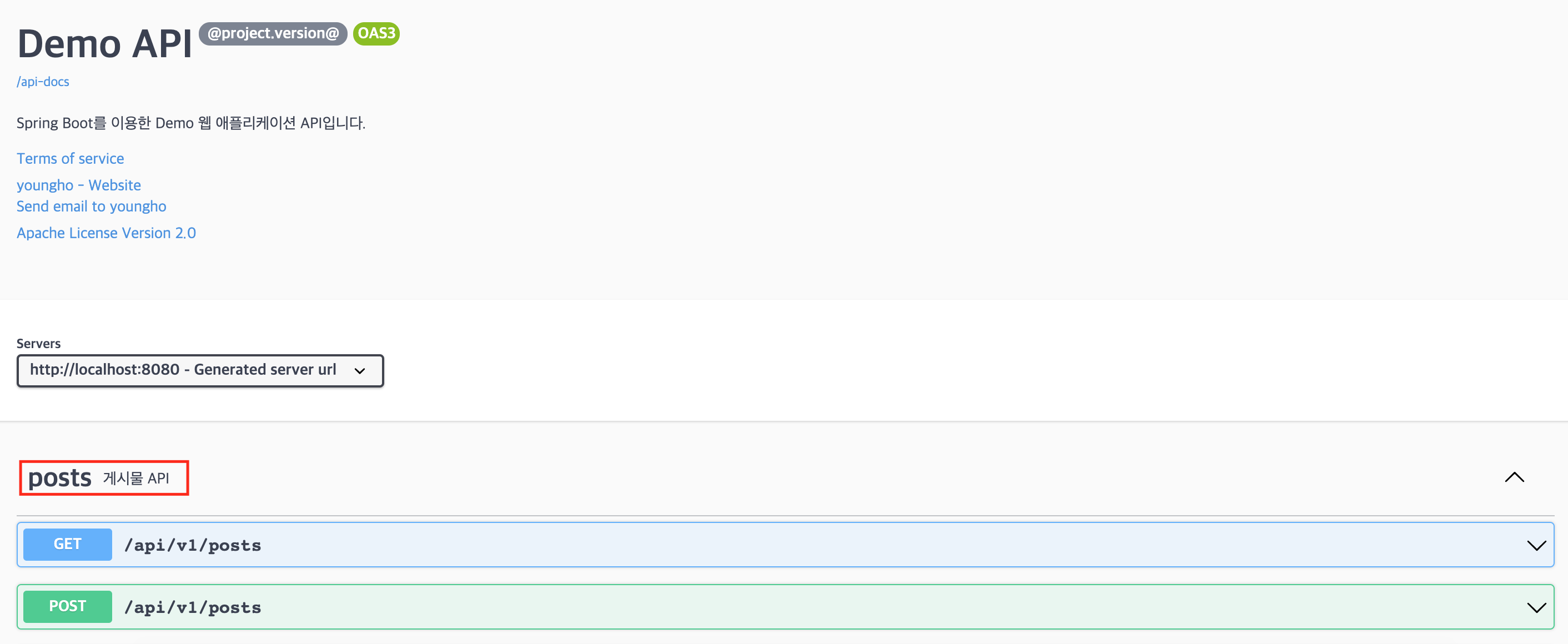
@Operation
- summary, security, descriptionโฆ
Example
1
2
3
| @Operation(summary = "get", description = "get user data by id")
@Operation(summary = "register", security = @SecurityRequirement(name = "bearer-token"))
|
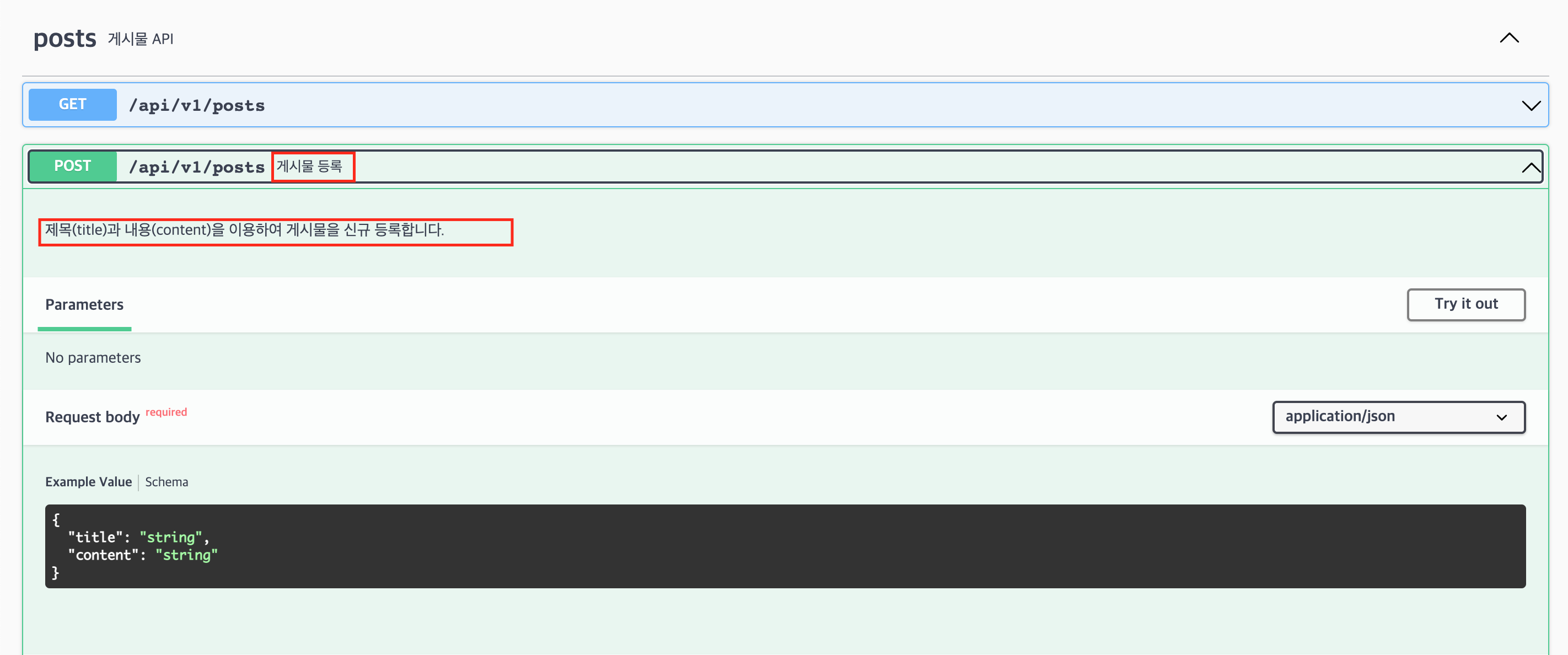
@Parameter
Example 1
- In @Parameters
1
2
3
| @Parameters({
@Parameter(name = "id", description = "์์ด๋")
})
|
Example 2
- In @Operations
1
2
3
4
| @Operation(parameters = {
@Parameter(name = "name", in = ParameterIn.QUERY,
schema = @Schema(implementation = String.class))
})
|
Example 3
- In method argument
1
2
3
4
5
6
7
8
9
10
11
12
13
14
| @Operation(summary = "๊ฒ์๊ธ ์กฐํ", description = "id๋ฅผ ์ด์ฉํ์ฌ posts ๋ ์ฝ๋๋ฅผ ์กฐํํฉ๋๋ค.",
responses = {
@ApiResponse(responseCode = "200", description = "๊ฒ์๊ธ ์กฐํ ์ฑ๊ณต",
content = @Content(schema = @Schema(implementation = PostsResponseDto.class))),
@ApiResponse(responseCode = "404", description = "์กด์ฌํ์ง ์๋ ๋ฆฌ์์ค ์ ๊ทผ",
content = @Content(schema = @Schema(implementation = ErrorResponse.class)))
})
@GetMapping("/posts/{id}")
public PostsResponseDto findById(@Parameter(name = "id",
description = "posts ์ id",
in = ParameterIn.PATH)
@PathVariable Long id) {
return postsService.findById(id);
}
|
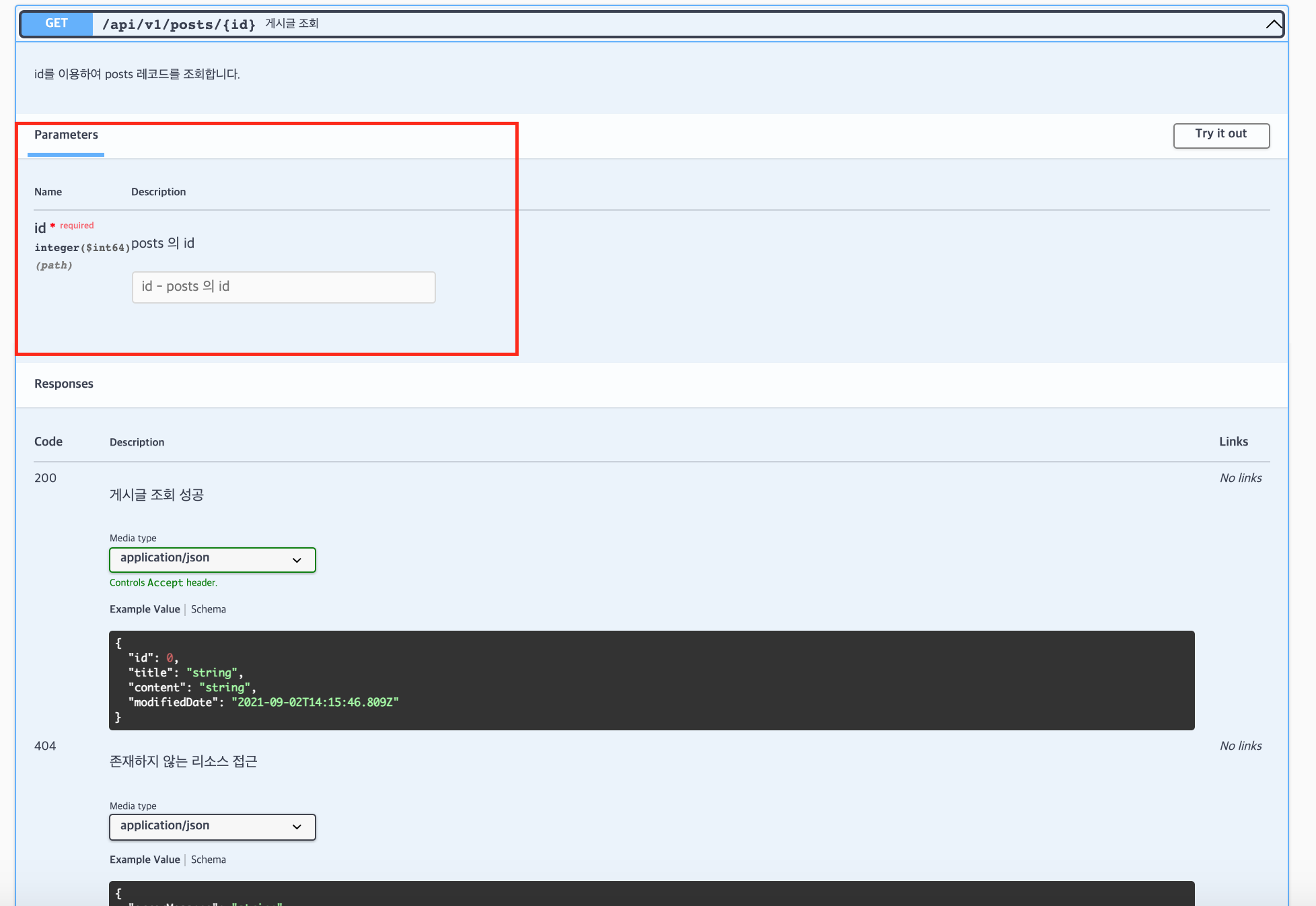
@ApiResponse
- description, responseCode, contentโฆ
- content : @Content, @ExampleObject, @ArraySchema, @Schemaโฆ
Use HTTP Status Code
Example
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
| // 200 OK
@ApiResponse(
description = "OK",
responseCode = "200",
content = {@Content(
array = @ArraySchema(
schema = @Schema(
implementation = SuccessInfoDto.class,
description = "detail"
)
)
)}
)
// 404 NOT FOUND
@ApiResponse(
description = "Not Found",
responseCode = "404",
content = {@Content(
examples = {
@ExampleObject(value =
"{\n" +
" \"timestamp\": \"2022-08-17\",\n" +
" \"error\": \"DataNotFound\",\n" +
" \"message\": \"There is no data\",\n" +
" \"status\": 404\n" +
"}"
)
}
)
}
)
|
Before | After |
---|
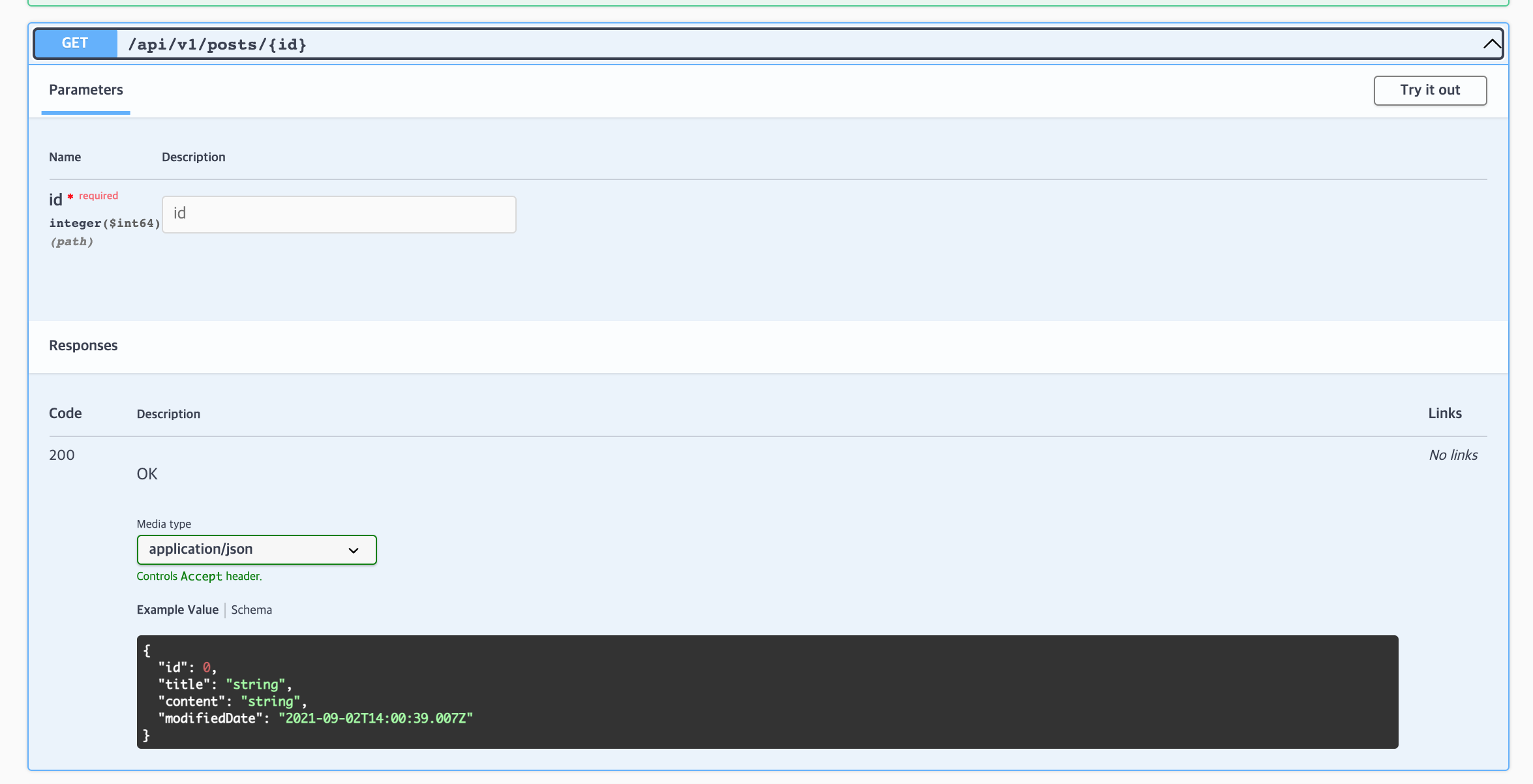 | 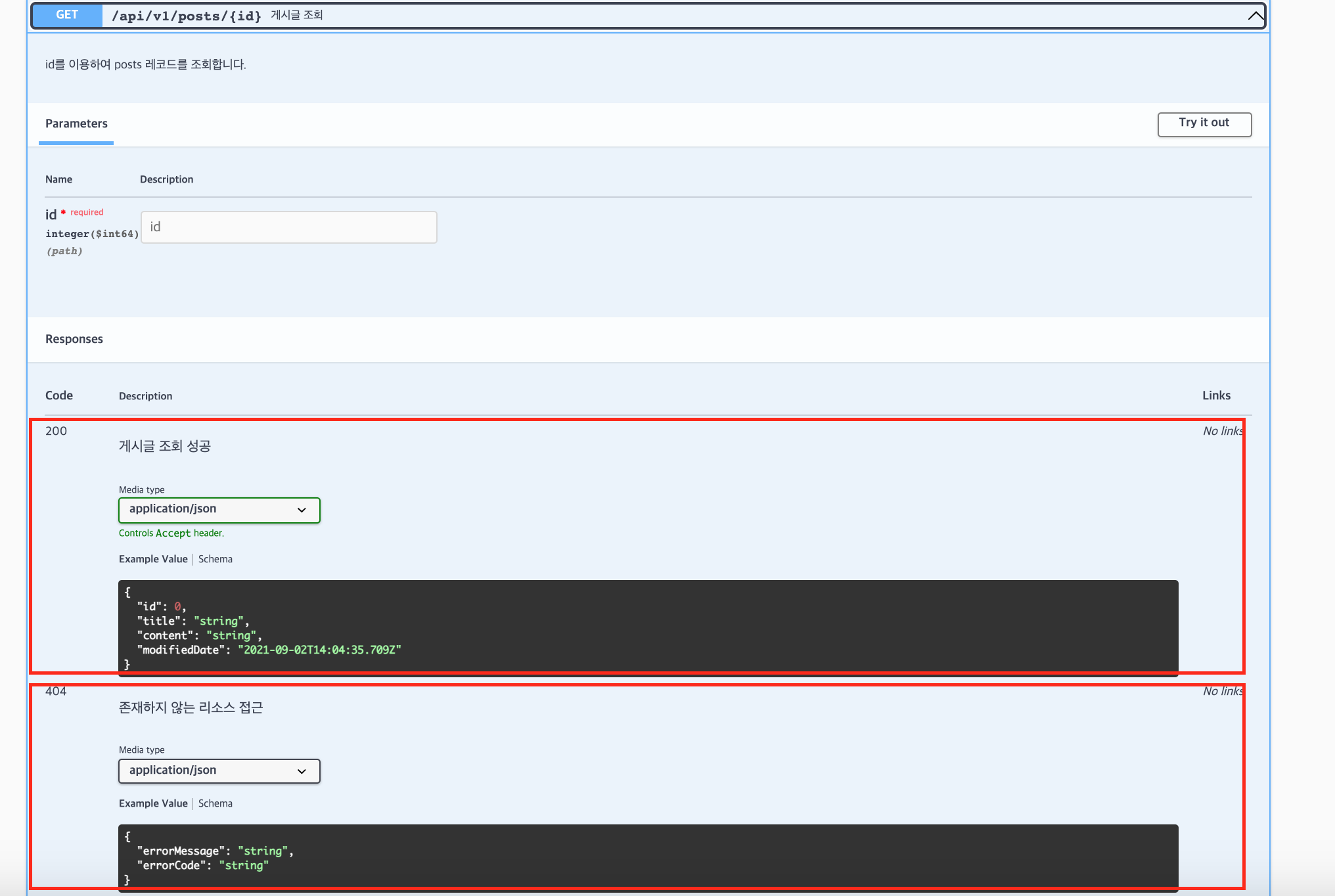 |
@Schema
- name, title, description, hiddenโฆ
Example 1
- in Dto.class
1
2
| @Schema(description = 'id', example = "1")
String id;
|
Example 2
- in @ApiResponse
1
2
3
4
5
| @ApiResponse(responseCode = "200",
description = "๊ฒ์๊ธ ์กฐํ ์ฑ๊ณต",
content = @Content(schema =
@Schema(implementation = PostsResponseDto.class))
)
|
Example 3
- in @Parameters
1
2
3
4
| @Parameters({
@Parameter(name = "id", description = "์์ด๋",
schema = @Schema(type = "integer", format = "int32", minimum = "1"))
})
|
Before | After |
---|
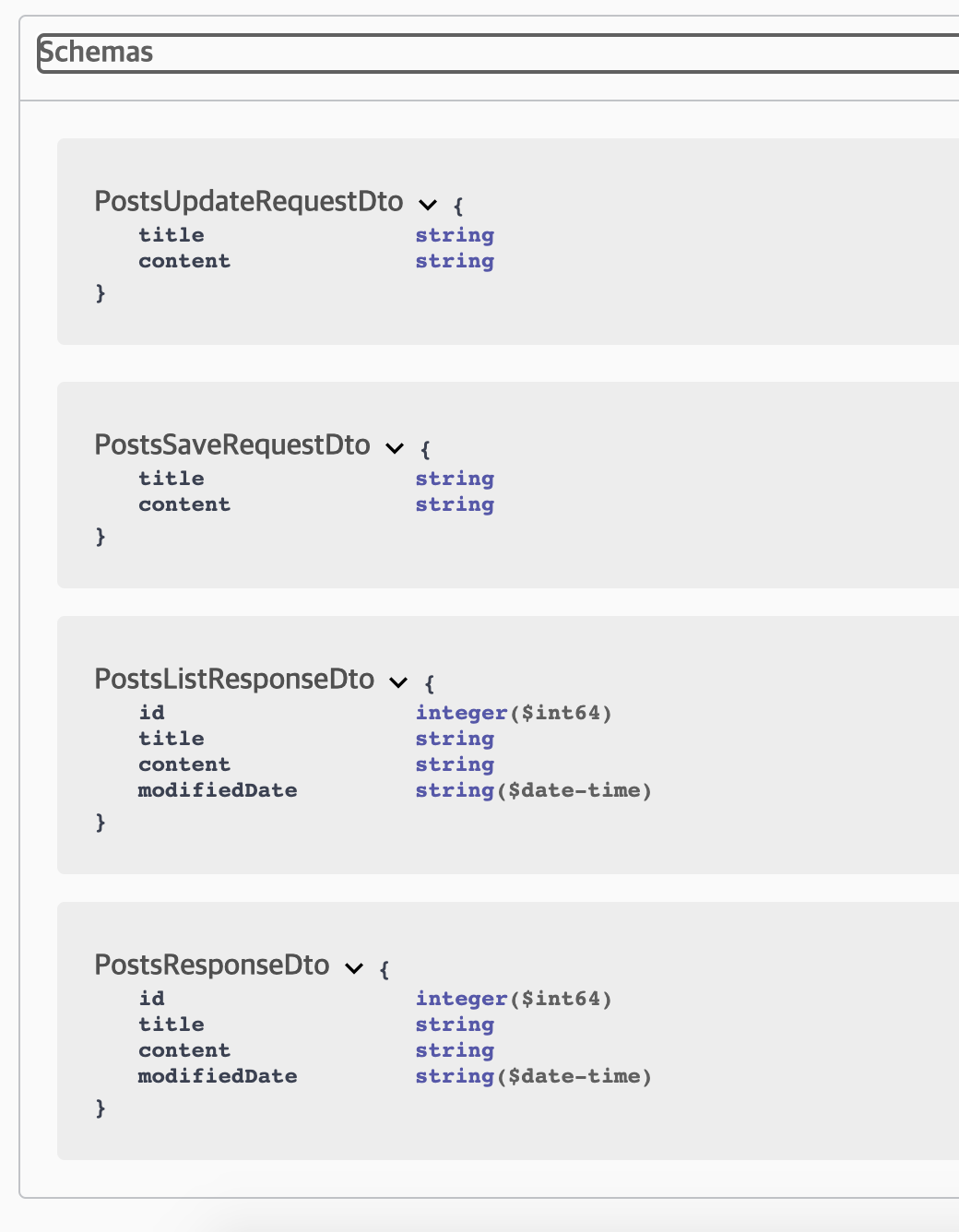 | 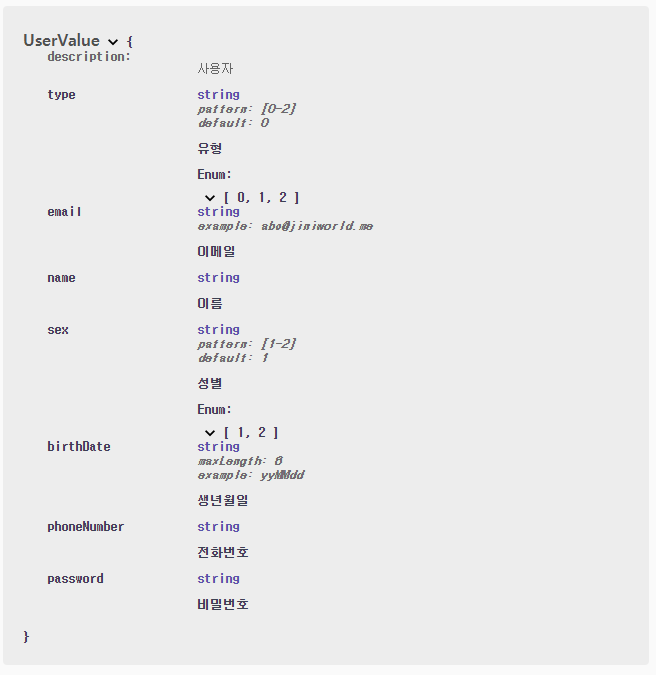 |
Code Example
Controller.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
| import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.Parameter;
import io.swagger.v3.oas.annotations.Parameters;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.tags.Tag;
@Tag(name = "Item", description = "ํ๋ชฉ")
@RestController
public class Controller {
@GetMapping(value = "/{id}")
@Operation(summary = "์กฐํ")
@Parameters({
@Parameter(name = "id", description = "์์ด๋")
})
@ApiResponse(
description = "",
responseCode = "200",
content = {
@Content(
schema = @Schema(
implementation = dto.class,
description = "detail"
)
)}
)
public ResponseEntity<Response> getResponse(@PathVariable String id) {
return ResponseEntity.of(service.findById(id));
}
}
|
Request.java
1
2
3
4
5
6
7
8
9
10
| import io.swagger.v3.oas.annotations.media.Schema;
public class Request {
@Schema(description = 'id', example = "1")
String id;
@Schema(hidden = true)
String status;
}
|
https://jeonyoungho.github.io/posts/Open-API-3.0-Swagger-v3/
https://velog.io/@hyejinjeong9999/springdoc%EC%9C%BC%EB%A1%9C-swagger3-%EB%8F%84%EC%9E%85
Comments powered by Disqus.